Clear Names for Complex Conditions
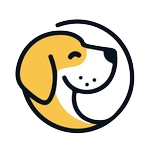
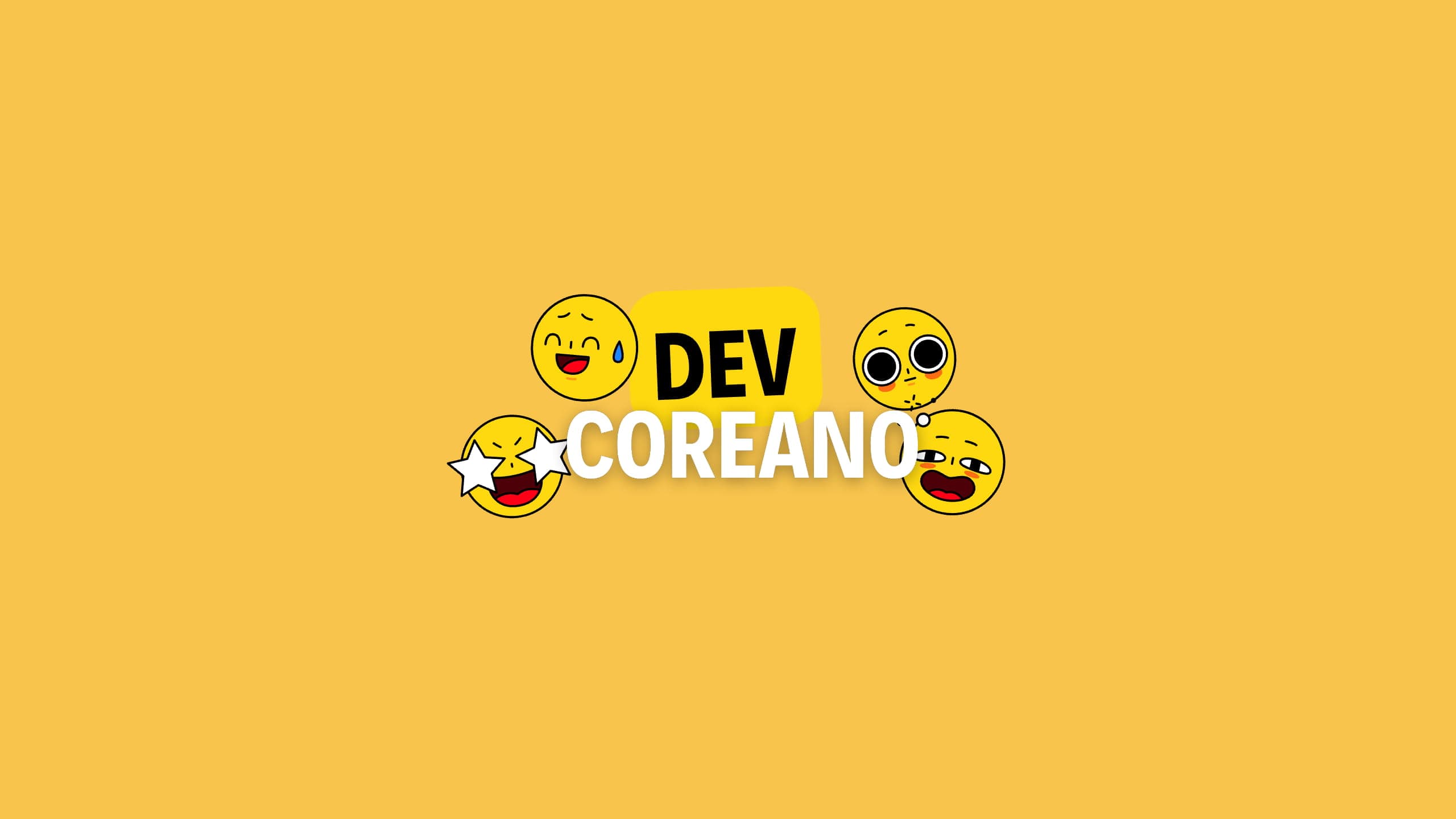
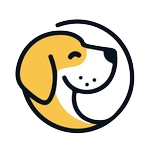
Complex conditions in code can be hard to read. Felienne Hermans said in her book "The Programmer's Brain" that we can remember only 6 things at once. So, dividing complex conditions into smaller ones can help us understand the code better. Let's see an example.
const result = users.filter((user) =>
user.permissions.some(
(permission) =>
permission.level >= requiredLevel &&
user.sessions.some(
(session) =>
session.lastActivity >= lastWeek &&
!session.isExpired
)
)
);
This code works. But it's hard to understand at a glance. Let's improve readability by breaking down the complex conditions into smaller parts.
const activeUsers = users.filter((user) => {
return user.permissions.some((permission) => {
const hasRequiredPermission = permission.level >= requiredLevel;
const hasRecentActivity = user.sessions.some((session) => {
const isRecent = session.lastActivity >= lastWeek;
const isValid = !session.isExpired;
return isRecent && isValid;
});
return hasRequiredPermission && hasRecentActivity;
});
});
I just named some parts of the logic. Dividing conditions into meaningful variables makes the code more readable. The first code shows us just the flow of logic. But the second one shows us both flow and meaning. For computers, they're the same. But for human developers, it's different. This shows us that adding meaningful context between the code is important.
Of course you don't need to name every part of the logic.
arr.map(x => x * 10 - 2)
This code is simple enough to understand at a glance. So, if you divide it, it might be overkill.
arr.map(x => {
const multiplied = x * 10;
const finalResult = multiplied - 2;
return finalResult;
});
But if you have a complex condition, it's better to name it.
Moreover, if you're working with a team where others need to use your code, or if you'll need to revisit your code after a long time, naming parts of the logic becomes crucial. It's like leaving notes for your future self or team members. I believe more than 80% of developers find themselves in this situation. So, just divide it and name it. It's worth it.